Sylian
Bannedbanned
LEVEL 28
56 XP
Welcome!
In this guide, I will show you how to make your own GM commands using the Eluna engine.
[NOTE]: I started on WoW emulation about 1 week ago now, so sorry if there are any errors!
[NOTE]: I strongly recommend you read the Eluna Engine Docs to learn more!
Step 1: Create a new lua file inside your folder called "lua_scripts" located inside your server folder.

Step 2: Open the new file you just created, when opened you should see an empty lua file like this.
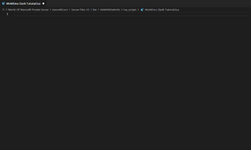
Step 3: Here we will program the whole command and then test it when we have it done, down below you can see the whole script, don't worry, I will explain it all so you can learn from it also
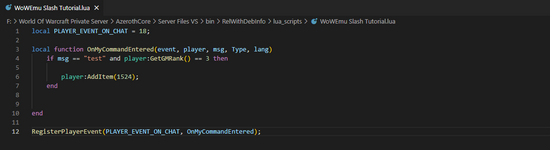
Let us start from the top!
On line 1 we create a new local variable that is called "PLAYER_EVENT_ON_CHAT" which is then set to "18", the reason we set it to "18" is because that is from my understanding the way wow emulation servers are setup with the events.
On line 3 we create a new local function with a name we can choose ourselves, as you might see there are some arguments (event, player, msg, Type, lang), these are the arguments that the "PLAYER_EVENT_ON_CHAT" returns when a player sends a chat message.
On line 4 we create an if statement where we first check if the player's message was equal to our command (in this case test), and then we check if the player that sent the message has a GM rank of 3 (That is the GameMaster rank), both of these are correct we will then enter the if statement contents.
If the command and GM rank was correct we will land on line 6 now, here we tell the script to give the player who sent the command an item (in this case we use an item with the ID 1524).
On the last line (12) we tell our script to "RegisterPlayerEvent", this means that our script will listen for the "PLAYER_EVENT_ON_CHAT" event, the other argument is what function it should call when the "PLAYER_EVENT_ON_CHAT" has been triggered.
That was all!
Let us go ahead and test it, shall we?

Congrats!
If everything is working right you should get the item called "Skullsplitter Tusk" when entering your new custom command!
If nothing happened, check this troubleshooting guide below!
1: Make sure you go to your World Server window and type ".reload eluna" and hit enter.
2: Make sure that your character is a GameMaster rank in your database.
4: Check the ElunaEngine Documentations if you want to see if you can fix it yourself!
3: Check your code again, if you need help you can hit me up in the PMs and I will see if I can help you!
In this guide, I will show you how to make your own GM commands using the Eluna engine.
[NOTE]: I started on WoW emulation about 1 week ago now, so sorry if there are any errors!
[NOTE]: I strongly recommend you read the Eluna Engine Docs to learn more!
Step 1: Create a new lua file inside your folder called "lua_scripts" located inside your server folder.

Step 2: Open the new file you just created, when opened you should see an empty lua file like this.
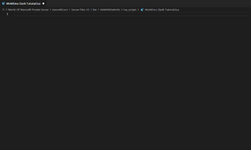
Step 3: Here we will program the whole command and then test it when we have it done, down below you can see the whole script, don't worry, I will explain it all so you can learn from it also
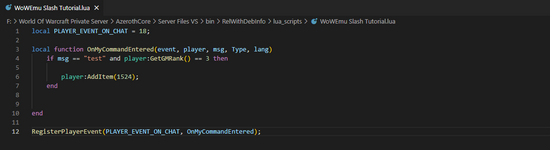
Let us start from the top!
On line 1 we create a new local variable that is called "PLAYER_EVENT_ON_CHAT" which is then set to "18", the reason we set it to "18" is because that is from my understanding the way wow emulation servers are setup with the events.
On line 3 we create a new local function with a name we can choose ourselves, as you might see there are some arguments (event, player, msg, Type, lang), these are the arguments that the "PLAYER_EVENT_ON_CHAT" returns when a player sends a chat message.
On line 4 we create an if statement where we first check if the player's message was equal to our command (in this case test), and then we check if the player that sent the message has a GM rank of 3 (That is the GameMaster rank), both of these are correct we will then enter the if statement contents.
If the command and GM rank was correct we will land on line 6 now, here we tell the script to give the player who sent the command an item (in this case we use an item with the ID 1524).
On the last line (12) we tell our script to "RegisterPlayerEvent", this means that our script will listen for the "PLAYER_EVENT_ON_CHAT" event, the other argument is what function it should call when the "PLAYER_EVENT_ON_CHAT" has been triggered.
That was all!
Let us go ahead and test it, shall we?

Congrats!
If everything is working right you should get the item called "Skullsplitter Tusk" when entering your new custom command!
If nothing happened, check this troubleshooting guide below!
1: Make sure you go to your World Server window and type ".reload eluna" and hit enter.
2: Make sure that your character is a GameMaster rank in your database.
4: Check the ElunaEngine Documentations if you want to see if you can fix it yourself!
3: Check your code again, if you need help you can hit me up in the PMs and I will see if I can help you!